Swing is a set if libraries in Java that provide the tools to build graphical forms. You get the libraries by adding this import:
import javax.swing.*;
Some of the layouts we will use such as the BorderLayout can be found in java.awt:
import java.awt.BorderLayout;
First we will look at the basics structure of a Swing form. The basic window is called a JFrame. The JFrame is the container for any windows content. Essentially it just forms a box. Here is some code that makes a basic JFrame and a picture of it running:
package com.spconger.SwingTests; import javax.swing.*; public class program { /** * @param args */ public static void main(String[] args) { // TODO Auto-generated method stub JFrame frame = new JFrame(); frame.setBounds(200,200,300,200); frame.setVisible(true); } }
Inside the JFrame it is most common to put one or more JPanel. JPanels are containers for controls such as labels, text boxes and buttons. We will look at three common layouts and then combine them in our simple hello world application. The types of layout are:
* FlowLayout
* GridLayout
* BorderLayout
Flow layout just flows the controls one after another a wraps at the form edges. It can be LEFT aligned or RIGHT aligned. Here is the code that adds a panel and uses flow layout. To illustrate the flow I have added JLabel controls. I also narrowed the JFrame width to 100 to show the flow wrap.
package com.spconger.SwingTests; import java.awt.FlowLayout; import javax.swing.*; public class program { /** * @param args */ public static void main(String[] args) { // TODO Auto-generated method stub program p = new program(); JFrame frame = new JFrame(); frame.setBounds(200,200,100,200); JPanel panel = p.createFlowLayoutPanel(); frame.add(panel); frame.setVisible(true); } private JPanel createFlowLayoutPanel(){ JPanel panel = new JPanel(); panel.setLayout(new FlowLayout(FlowLayout.LEFT)); JLabel lblOne=new JLabel("ONE"); JLabel lblTwo=new JLabel("TWO"); JLabel lblThree=new JLabel("THREE"); JLabel lblFour=new JLabel("FOUR"); panel.add(lblOne); panel.add(lblTwo); panel.add(lblThree); panel.add(lblFour); return panel; }
Here is a picture of the form.
Next we will do a grid layout. For a grid layout you set up the rows and the columns you want. You don't have control over which grid cell a control goes into really. They just flow first control, first cell, second goes into the second cell, left to right, etc.
package com.spconger.SwingTests; import java.awt.FlowLayout; import java.awt.GridLayout; import javax.swing.*; public class program { /** * @param args */ public static void main(String[] args) { // TODO Auto-generated method stub program p = new program(); JFrame frame = new JFrame(); frame.setBounds(200,200,100,200); JPanel panel = p.createGridLayoutPanel(); frame.add(panel); frame.setVisible(true); } private JPanel createGridLayoutPanel(){ JPanel panel = new JPanel(); //this is the only line that is different panel.setLayout(new GridLayout(2,2)); JLabel lblOne=new JLabel("ONE"); JLabel lblTwo=new JLabel("TWO"); JLabel lblThree=new JLabel("THREE"); JLabel lblFour=new JLabel("FOUR"); panel.add(lblOne); panel.add(lblTwo); panel.add(lblThree); panel.add(lblFour); return panel; } }
Here is a picture of the form with the grid layout
The border layout we will use in context with our hello world app. The border layout sets designations for the different areas of the panel. The top is NORTH, the botton SOUTH, Left is EAST and Right is WEST. The center has a CENTER designation. You can use the border layout of a panel to help arrange other panels.
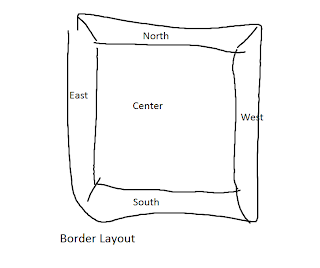
What we are going to do, is create a panel with a border layout. We will add a grid layout in the center that has a JLabel, a aJTextField and another JLabel
Then we will add a JPanel to the SOUTH. It will have a flow layout and contain two JButtons.
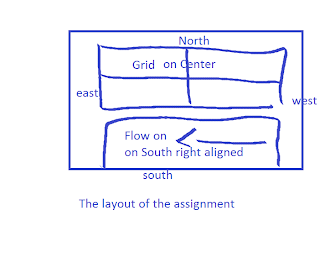
What the program will do is take a user's name as in a JTextField. When the user clicks the JButton btnSubmit. the second JLabel will display a greeting. A second JButton, btnExit, will exit the program. To handle the buttons we will need to add Action listeners. Will will do this by implementing the ActionListeners interface. Later I will show how to do listeners as internal classes.
Here is the full code. I have commented it to help clarify what is going on.
package com.spconger.FirstSwing; import javax.swing.*; import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; //the class implements the interface ActionListener public class Program implements ActionListener{ JButton btnExit; JButton btnGetName; JLabel result; JTextField txtName; /** * Build through this one very slowly in class * first show flow layout then grid * build up the panel, add one thing at a time * until we arrive at this form * talk about frame and panel and controls * and then the listeners. * maybe reorganize the methods to have more logic * and declare all the elements at top. * @param args */ public static void main(String[] args){ //instantiate the non static parts of the class Program p = new Program(); //call the createFrame method p.createFrame(); } private void createFrame(){ JFrame frame = new JFrame(); //frame.setDefaultCloseOperation(EXIT_ON_CLOSE)); frame.add(createPanel()); frame.setBounds(200,200,300,150); frame.setVisible(true); } private JPanel createPanel(){ //this method creates the main panel which //has a border layout //then it calls and adds the grid and flow layout //panels JPanel mainPanel=new JPanel(); mainPanel.setLayout(new BorderLayout()); mainPanel.add(createInfoPanel(),BorderLayout.CENTER); mainPanel.add(createButtonPanel(), BorderLayout.SOUTH); return mainPanel; } private JPanel createInfoPanel(){ //this creates a new grid layout panel JPanel panel = new JPanel(); //the grid has two rows and 2 columns with a 5 pixel space //between them panel.setLayout(new GridLayout(2,2,5,5)); //add the controls JLabel label = new JLabel("Enter You name"); panel.add(label); txtName = new JTextField(); panel.add(txtName); result = new JLabel(); panel.add(result); return panel; } private JPanel createButtonPanel(){ //the button panel is a flow layout panel JPanel buttonPanel =new JPanel(); buttonPanel.setLayout(new FlowLayout(FlowLayout.RIGHT)); btnGetName=new JButton("Submit"); //each button needs to be directed to the action listener btnGetName.addActionListener(this); buttonPanel.add(btnGetName); btnExit = new JButton("Exit"); btnExit.addActionListener(this); buttonPanel.add(btnExit); return buttonPanel; } @Override //this method handles the button click events //for both buttons public void actionPerformed(ActionEvent e) { // TODO Auto-generated method stub Object source = e.getSource(); if(source==btnExit){ System.exit(0); } if (source==btnGetName){ result.setText("Hello, " + txtName.getText()); } } }
Now here is a picture of the code running after the name has been entered and the submit button clicked
No comments:
Post a Comment