Here is the UML diagram
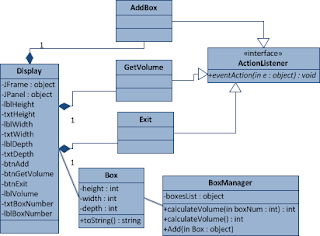
I have also posted this on Git Hub. Also look at the comment to this blog to see a correction to the code offered by Mitchel
Here is the Box class
package com.spconger.Boxes; public class Box { private int height; private int width; private int depth; public void setHeight(int height){ this.height=height; } public int getHeight(){ return height; } public int getWidth() { return width; } public void setWidth(int width) { this.width = width; } public int getDepth() { return depth; } public void setDepth(int depth) { this.depth = depth; } @Override public String toString() { // TODO Auto-generated method stub return "height: " + getHeight() + ", width: " + getWidth() + ", depth: " + getDepth(); } }
Here is the BoxManager class
package com.spconger.Boxes; import java.util.ArrayList; public class BoxManager { private ArrayListboxList; public BoxManager(){ boxList=new ArrayList (); } public void add(Box b){ boxList.add(b); } public int getVolume(){ int volume=0; int h=0, w=0, d=0; for(Box b:boxList){ h = b.getHeight(); w = b.getWidth(); d = b.getDepth(); volume += h * w * d; } //volume=h * w * d; return volume; } public int getVolume(int boxNumber){ int volume=0; if (boxNumber <= boxList.size()){ Box b = boxList.get(boxNumber); volume =b.getDepth() * b.getHeight() * b.getWidth(); } return volume; } }
Here is the MainForm class
package com.spconger.Boxes; import javax.swing.*; import java.awt.GridLayout; import java.awt.event.*; public class MainForm { //declare all the form elements private JFrame frame; private JPanel panel; private JLabel lblHeight; private JTextField txtHeight; private JLabel lblWidth; private JTextField txtWidth; private JLabel lblDepth; private JTextField txtDepth; private JLabel lblVolume; private JButton btnAdd; private JButton btnGetVolume; private JTextField txtBoxNumber; private JButton btnExit; private BoxManager bxManager; public MainForm(){ //constructor // createFrame(); bxManager=new BoxManager(); } //create the frame private void createFrame(){ frame = new JFrame(); frame.setSize(300,300); createPanel(); frame.add(panel); frame.setVisible(true); } //create the panel private void createPanel(){ panel = new JPanel(); panel.setLayout(new GridLayout(6,2,5,5)); //make all the objects new lblHeight=new JLabel("Enter the Height"); txtHeight=new JTextField(); lblWidth=new JLabel("Enter the Width"); txtWidth=new JTextField(); lblDepth=new JLabel("Enter the Depth"); txtDepth=new JTextField(); btnAdd = new JButton("Add"); btnAdd.addActionListener(new AddBox()); btnGetVolume = new JButton("Get Volume"); btnGetVolume.addActionListener(new GetBoxVolumes()); txtBoxNumber = new JTextField(); btnExit = new JButton("Exit"); btnExit.addActionListener(new ExitEvent()); lblVolume = new JLabel(); //add them all to the panel panel.add(lblHeight); panel.add(txtHeight); panel.add(lblWidth); panel.add(txtWidth); panel.add(lblDepth); panel.add(txtDepth); panel.add(btnAdd); panel.add(btnGetVolume); panel.add(txtBoxNumber); panel.add(btnExit); panel.add(lblVolume); } //private classes to implement button actions private class ExitEvent implements ActionListener{ @Override public void actionPerformed(ActionEvent arg0) { System.exit(0); } } private class AddBox implements ActionListener{ @Override public void actionPerformed(ActionEvent e) { Box b = new Box(); b.setWidth(Integer.parseInt(txtWidth.getText())); b.setDepth(Integer.parseInt(txtDepth.getText())); b.setHeight(Integer.parseInt(txtHeight.getText())); bxManager.add(b); txtHeight.setText(""); txtWidth.setText(""); txtDepth.setText(""); } } private class GetBoxVolumes implements ActionListener{ @Override public void actionPerformed(ActionEvent e) { int volume=0; //if (txtBoxNumber.getText() != null || !txtBoxNumber.getText().equals("")){ // int boxIndex=Integer.parseInt(txtBoxNumber.getText()); //volume=bxManager.getVolume(boxIndex); //} // else //{ volume=bxManager.getVolume(); //} lblVolume.setText("the volume is: " + volume); } } }
Here is the Program class
package com.spconger.Boxes; public class Program { /** * @param args */ public static void main(String[] args) { //call main form that creates form MainForm form = new MainForm(); } }
Hey Steve, I found the problem with doing both for the if/else on the "all" of them issue, or none.
ReplyDeleteThe if should be trying to catch an empty string, and then let the else deal with the numbers. Here's the working code for GetBoxVolumes():
private class GetBoxVolumes implements ActionListener{
@Override
public void actionPerformed(ActionEvent arg0) {
int volume = 0;
if (txtBoxNumber.getText().equals( "" )){
volume=bxManager.getVolume();
}
else{
int boxIndex=Integer.parseInt(txtBoxNumber.getText());
volume = bxManager.getVolume(boxIndex);
}