community Assist application
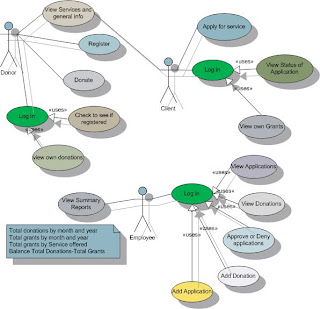
#include
using namespace std;
void ConvertInches(int inches);
int main()
{
cout << "Please Enter your height in inches." << endl;
int inch;
cin << inch;
ConvertInches(inch);
char c;
cin << c;
}
void ConvertInches(int inches)
{
const int FOOT=12;
int heightInFeet = inches / FOOT;
int remainingInches = inches % FOOT;
cout << "you are " << heightInFeet << " feet and "
<< remainingInches << " inches tall." << endl;
}
Here is the code for the arrays
#include
#include//add the string library
using namespace std;
int main()
{
const int size =5; //declare a constant
int myArrayb[size]; //arrays require a constant for limit
int myArray[4] = {4,7,23,16}; //initialize the array
char name[]="George Jetson";//character array
//this gets the character array and the length of
//the character array and then choses the fifth character
//from the array
cout << "Your name is "<< name << "there are "
<< strlen(name) << " characters in your name"
<< "The fifth character is " << name[4] <<
endl;
//gets the third element from the first array
cout << "the 3rd element is " << myArray[2] << endl;
//use of getline with a character array
cout << "enter your first and last name" << endl;
char fullName[25];
cin.getline(fullName, 25);
string address; //declare a variable as string
cout << "Enter your address" << endl;
//use string getline
getline(cin, address);
cout << "Your name is " << fullName << endl;
cout << "Your address is " << address << endl;
//just pause the program waiting for a character
//to be entered
char c;
cin << c;
}
#include <iostream>
#include <string>
#include <cmath>
using namespace std;
int cube(int); //function prototype
int main()
{
/* this is a simple hello application
the comment can cross
several lines */
cout << "Enter your name" << endl;
char name[25];
cin.getline(name, 25);
cout << "Hello," << name << endl;
cout << "Enter a number" << endl;
int num;
cin >> num;
cout << "the cube of " << num << " is " << cube(num) << endl;
cout << "The square root of " << num << " is " << sqrt((double)num) << endl;
//this is just to pause the console
char c; //declare a character type variable
cin >> c; //wait for console entry
}
int cube (int x)
{
return x * x * x;
}